Paneler Script
Friday, September 29th 2023
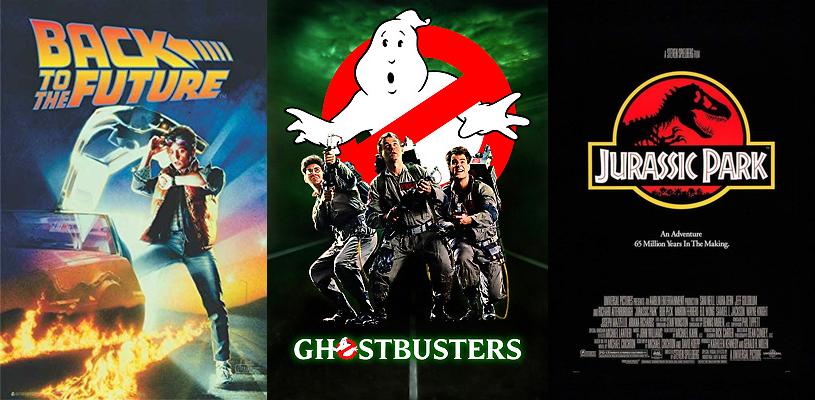
Every now and then, you find yourself doing a repetitive task. After about the third or four time, you're like "I can automate this." I find myself doing this all the time. Sometimes I can find ways to automate, sometimes I'm just out of luck. But this time I was able to. So I thought I would share this script I wrote and have found useful. Let me try to explain what it does.
The ProblemI write another blog about tv and movie cars. Every so often, I do post about upcoming streaming offerings of movies and tv shows that feature cars. I like to feature the posters of the movies in the post as a panel. Or at least that's the only terminology I can think of to describe it. You can see an example of this above. If you can think of a better name or know the proper name. Let me know! For years, I would throw the posters into MS-Paint. I would find the smallest poster by height and then add them all manually. For a three or four poster panel this would take me a few minutes. I finally said, enough is enough. Let's automate this. But how?
The SolutionFor the last year or so, I have been studying Python. Just so happen to stumble across a Python library called Pillow. This library is just for working with images. It can do all kinds of stuff! So after thinking through the steps I would have done in MS-Paint, I was able to recreate that process in a Python script. Feel free to checkout the result below and copy or use it for your own use. As always, no warranties and we assume no reasonability for damages.
# Name: paneler # Description: Merges all of the images in the 'files' directory into a single inline panel image. import os from PIL import Image dir = "files" files = os.listdir(dir) smallestHeight = None # load images into an list images = [] for idx, file in enumerate(files): images.append(Image.open(f"{dir}/{file}")) # track the smallest height if smallestHeight == None or images[idx].height < smallestHeight: smallestHeight = images[idx].height newWidth = 0 newHeight = smallestHeight # resize all other images to match smallest height for idx, image in enumerate(images): if image.width != smallestHeight: image.thumbnail(size=(images[idx].width, smallestHeight), resample=Image.Resampling.LANCZOS) newWidth += images[idx].width # build a new image newImage = Image.new(mode="RGB", size=(newWidth, newHeight), color=(0,0,255)) left = 0 # add images to new image, increase left by current width for idx, image in enumerate(images): newImage.paste(image, (left, 0)) left += image.width newImage.save(f"{dir}/newimage.jpg")
Categories: Development, Programming, Tools
Tags: python